The world is moving fast toward real-time artificial intelligence, where decisions are made in milliseconds, and machines can understand visual data just like humans.
One of the core technologies enabling this future is PyTorch, an open-source machine learning framework that’s powering next-gen image recognition systems — from autonomous vehicles to live surveillance to real-time augmented reality.
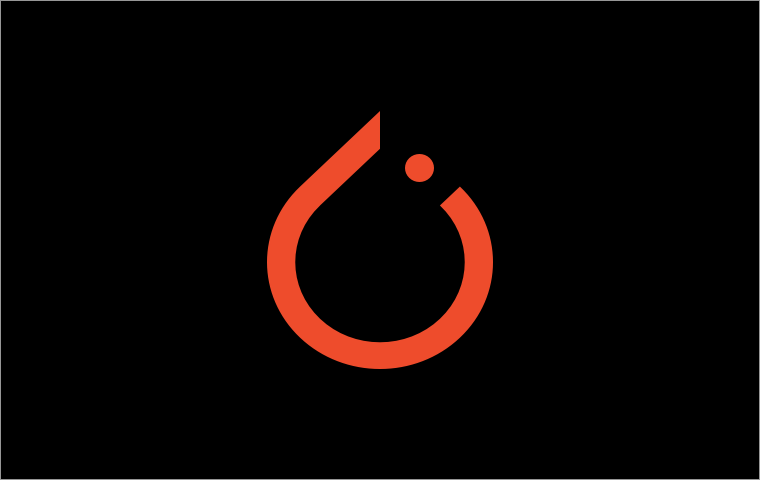
In this article, we’ll walk you through getting started with PyTorch for image recognition, including what makes it ideal for real-time applications, how to build your first computer vision model, and tips for deploying it at scale.
Table of Contents
What Is PyTorch and Why It Matters in Real-Time AI?
PyTorch is an open-source deep learning framework developed by Meta AI (formerly Facebook AI). It offers:
- A dynamic computational graph, allowing intuitive and flexible coding
- A large ecosystem of pre-trained models and tools for computer vision
- Native integration with CUDA for GPU acceleration
- Strong support for research and production deployments
In real-time applications, where speed and accuracy are critical, PyTorch provides the speed of TensorFlow with the flexibility of Python — a balance that’s crucial for image recognition tasks.
What Is Real-Time Image Recognition?
Image recognition is the process by which a computer system identifies objects, people, places, or actions in an image. When this happens in real time, the model must:
- Process frames from a camera feed instantly
- Predict with high accuracy and low latency
- Scale efficiently across devices (cloud, edge, mobile)
PyTorch, combined with powerful libraries like TorchVision and TorchServe, makes this not only possible but increasingly accessible.
Popular Real-Time Image Recognition Use Cases
Application | Example Use Case |
---|---|
Healthcare | Detecting tumors in X-rays during live screening |
Retail | Shelf monitoring via smart cameras |
Security | Real-time facial recognition and intruder alerts |
AR/VR | Object recognition for dynamic overlays |
Self-driving cars | Road sign and pedestrian detection |
All of these systems rely on PyTorch or similar frameworks to process live image data and respond in real time.
Setting Up PyTorch for Image Recognition
Step 1: Install PyTorch
Visit https://pytorch.org to generate the correct installation command for your environment.
Basic installation via pip:
bashCopiarEditarpip install torch torchvision torchaudio
For GPU acceleration:
bashCopiarEditarpip install torch torchvision torchaudio --index-url https://download.pytorch.org/whl/cu118
Step 2: Load a Pre-trained Image Recognition Model
pythonCopiarEditarimport torch
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
# Load a pre-trained model (e.g., ResNet18)
model = models.resnet18(pretrained=True)
model.eval()
Step 3: Prepare an Input Image
pythonCopiarEditartransform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
image = Image.open("sample.jpg")
input_tensor = transform(image).unsqueeze(0)
Step 4: Make a Prediction
pythonCopiarEditarwith torch.no_grad():
output = model(input_tensor)
predicted_class = output.argmax().item()
print("Predicted class index:", predicted_class)
You can map the class index to human-readable labels using ImageNet class mappings from TorchVision.
Making It Real-Time: Frame-by-Frame Video Analysis
To process video frames from a live camera feed:
pythonCopiarEditarimport cv2
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
image = Image.fromarray(cv2.cvtColor(frame, cv2.COLOR_BGR2RGB))
input_tensor = transform(image).unsqueeze(0)
with torch.no_grad():
output = model(input_tensor)
pred = output.argmax().item()
cv2.putText(frame, f"Class: {pred}", (10, 30), cv2.FONT_HERSHEY_SIMPLEX,
1, (0, 255, 0), 2, cv2.LINE_AA)
cv2.imshow("Real-Time Recognition", frame)
if cv2.waitKey(1) & 0xFF == ord("q"):
break
cap.release()
cv2.destroyAllWindows()
This simple script enables live prediction using a webcam and PyTorch’s inference pipeline.
Why PyTorch Is Ideal for Real-Time Image Recognition
Feature | Benefit |
---|---|
Dynamic computation graph | Enables real-time debugging and input flexibility |
Native GPU acceleration | Fast processing of high-resolution frames |
Pre-trained models | Reduces time to deploy working prototypes |
Mobile and edge support | Convert models to TorchScript or ONNX for deployment |
Active ecosystem | Supported by libraries like TorchVision, FastAI, Detectron2 |
Deploying PyTorch Models in Production
To deploy your PyTorch image recognition model:
🔸 Use TorchServe
- A model serving framework built specifically for PyTorch
- Supports REST APIs, model versioning, metrics, and batch inference
bashCopiarEditartorch-model-archiver --model-name resnet18 --version 1.0 \
--model-file model.py --serialized-file model.pth \
--handler image_classifier
🔸 Export to ONNX
- For cross-platform deployment, including mobile and browser-based apps
pythonCopiarEditartorch.onnx.export(model, input_tensor, "model.onnx")
Advanced Projects with PyTorch and Image Recognition
Once you’re comfortable with basic classification, you can explore:
- Object detection (e.g., YOLOv5 in PyTorch)
- Segmentation (e.g., Mask R-CNN with Detectron2)
- Face recognition (e.g., FaceNet + PyTorch implementation)
- Custom training with your own dataset using DataLoader and transfer learning
Tips for Beginners in PyTorch + Vision
- Use GPU when possible — CPU is much slower for inference
- Start with small datasets and fine-tune pre-trained models
- Learn to visualize model predictions to better understand accuracy
- Follow tutorials on Kaggle, PyTorch official docs, and FastAI
- Use Google Colab or AWS Sagemaker for cloud-based training
PyTorch in the Real World
📱 Meta’s AI camera systems
Use PyTorch for gesture and object recognition in AR experiences.
🚗 Tesla and autonomous driving startups
Implement image recognition models trained in PyTorch for real-time obstacle detection.
🏥 Healthcare companies
Use PyTorch-based models for analyzing X-rays, MRIs, and even retina scans.
Conclusion: PyTorch Powers the Future of Real-Time Image Recognition
As real-time AI becomes the new normal, the need for fast, efficient, and customizable deep learning frameworks grows. PyTorch stands at the center of this transformation, offering developers the tools they need to build the next generation of vision-based AI.
Whether you’re a hobbyist building a webcam classifier or a startup founder deploying scalable image recognition at the edge — getting started with PyTorch today means preparing your AI stack for the demands of tomorrow.
Sources That Inspired This Article
- PyTorch Documentation
- Meta AI Research Blog
- OpenCV and TorchVision GitHub Repositories
- FastAI Tutorials
- Papers With Code: Image Classification Benchmarks
- PyImageSearch – Real-Time Image Recognition with PyTorch
Website: https://4news.tech
Email: [email protected]