In today’s digital world, language is at the heart of how we interact with technology. From chatbots to voice assistants and language translation apps, natural language processing (NLP) is transforming the way machines understand human communication. And one of the most powerful tools helping developers build these intelligent systems is PyTorch.
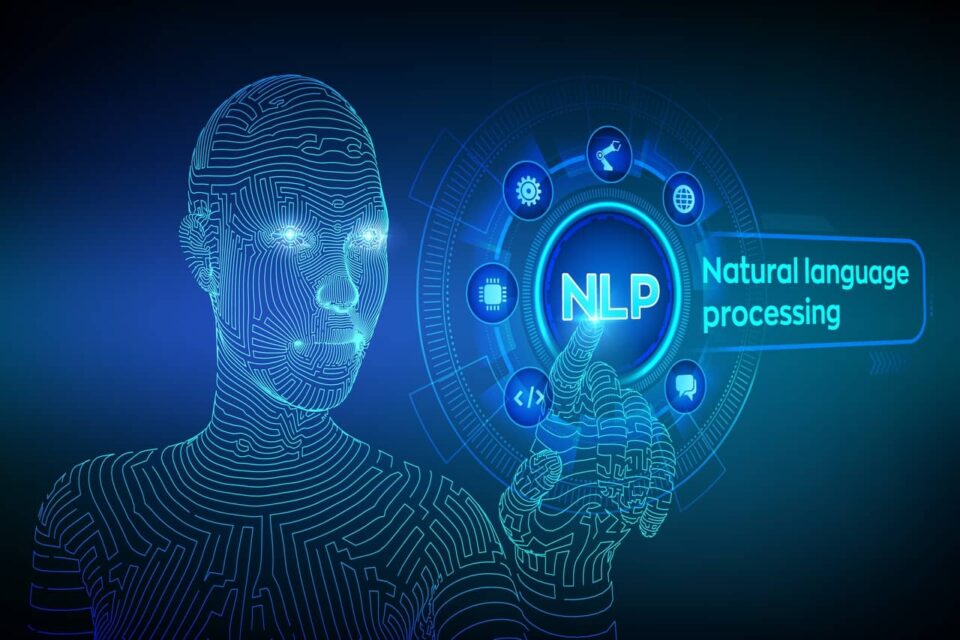
In this guide, we’ll walk you through the basics of using PyTorch for NLP — what makes it special, how to build your first text-based model, and where to go next as you grow your skills in deep learning and language understanding.
Table of Contents
What Is PyTorch?
PyTorch is an open-source machine learning framework developed by Meta AI (formerly Facebook). It’s become one of the most popular platforms for building deep learning models thanks to:
- Dynamic computation graphs, making model development more intuitive
- Strong community support and integration with popular libraries
- Powerful GPU acceleration for training large models
- Native Python compatibility, making it easier to learn for beginners
While PyTorch is widely used for computer vision, it’s also a top choice for NLP — powering applications like translation, summarization, classification, and chatbot development.
Why Use PyTorch for Natural Language Processing?
Benefit | How It Helps in NLP |
---|---|
Dynamic graph computation | Allows flexible model design for sequential data |
Built-in tokenizers and embeddings | Simplifies text preprocessing workflows |
Support for RNNs, LSTMs, and Transformers | Covers all modern NLP architectures |
TorchText & Hugging Face integration | Easy access to datasets and pre-trained models |
Active NLP research community | Constant updates and tutorials available |
Whether you’re analyzing tweets, building a chatbot, or training your own language model, PyTorch provides the tools to do it effectively and efficiently.
Key NLP Concepts You Should Know Before Starting
Before building in PyTorch, it’s helpful to understand a few key NLP basics:
- Tokenization – Breaking text into words or subwords
- Embedding – Representing words as numerical vectors
- Sequence modeling – Processing text in order (e.g., for prediction)
- Classification – Labeling text (e.g., spam detection, sentiment analysis)
- Transformer models – State-of-the-art architecture for NLP tasks
Don’t worry — PyTorch makes it easy to work with these concepts.
Getting Started with PyTorch for NLP
Let’s walk through a simple NLP project using PyTorch: Text Classification (e.g., detecting sentiment in a movie review).
Step 1: Install PyTorch
bashCopiarEditarpip install torch torchvision torchaudio
(Optional but recommended for NLP work)
bashCopiarEditarpip install torchtext transformers
Step 2: Import Libraries
pythonCopiarEditarimport torch
import torch.nn as nn
import torch.optim as optim
from torchtext.datasets import AG_NEWS
from torchtext.data.utils import get_tokenizer
from torchtext.vocab import build_vocab_from_iterator
Step 3: Load and Tokenize the Data
pythonCopiarEditartokenizer = get_tokenizer('basic_english')
def yield_tokens(data_iter):
for label, line in data_iter:
yield tokenizer(line)
train_iter = AG_NEWS(split='train')
vocab = build_vocab_from_iterator(yield_tokens(train_iter), specials=["<unk>"])
vocab.set_default_index(vocab["<unk>"])
Step 4: Define the Model
pythonCopiarEditarclass TextClassifier(nn.Module):
def __init__(self, vocab_size, embed_dim, num_class):
super().__init__()
self.embedding = nn.Embedding(vocab_size, embed_dim)
self.fc = nn.Linear(embed_dim, num_class)
def forward(self, text):
embedded = self.embedding(text)
return self.fc(embedded.mean(0))
vocab_size = len(vocab)
embed_dim = 64
num_class = len(set([label for label, _ in AG_NEWS(split='train')]))
model = TextClassifier(vocab_size, embed_dim, num_class)
Step 5: Train the Model
pythonCopiarEditarcriterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=4.0)
def train_loop(dataloader):
model.train()
for label, text in dataloader:
optimizer.zero_grad()
predicted = model(text)
loss = criterion(predicted, label)
loss.backward()
optimizer.step()
Step 6: Evaluate Performance
Use a test set and measure accuracy with standard metrics such as precision, recall, and F1-score. You can also visualize confusion matrices to improve classification performance.
Popular NLP Projects You Can Build with PyTorch
Project | Description |
---|---|
Sentiment Analyzer | Classify tweets or reviews as positive/negative |
Text Summarizer | Generate concise summaries of long documents |
Chatbot with Transformers | Build a smart conversational agent |
Named Entity Recognition (NER) | Highlight people, places, organizations in text |
Machine Translation | Translate text between languages |
Using Transformers and Hugging Face with PyTorch
In 2025, most NLP tasks are dominated by Transformer-based models like BERT, GPT, and RoBERTa. Luckily, PyTorch works seamlessly with the Hugging Face Transformers library.
Example: Sentiment Analysis with DistilBERT
pythonCopiarEditarfrom transformers import pipeline
classifier = pipeline("sentiment-analysis", model="distilbert-base-uncased-finetuned-sst-2-english")
print(classifier("I love using PyTorch for NLP!"))
This example loads a pre-trained PyTorch model that gives sentiment classification out of the box.
Best Practices for NLP with PyTorch
- Use GPU acceleration for training large models (with
.cuda()
orto(device)
) - Leverage batch processing and DataLoaders for speed and scalability
- Fine-tune pre-trained models for specific tasks (e.g., domain-specific language)
- Use tensorboard or Weights & Biases for experiment tracking
- Always evaluate models with real-world examples and avoid overfitting
Real-World Applications of PyTorch in NLP
Industry | Application Example |
---|---|
Healthcare | Automating medical report summarization |
Legal | Document classification and case tagging |
Finance | News sentiment for market predictions |
E-commerce | Product review analysis and chatbot automation |
Education | Automated essay scoring and tutoring systems |
PyTorch is at the core of AI platforms built by companies like Meta, Microsoft, and Hugging Face — and it’s powering NLP at scale across the globe.
What’s Next in PyTorch and NLP?
Looking into the future of AI and NLP:
- Multilingual models will become standard in global apps
- Real-time NLP will power voice assistants and AR interfaces
- Low-resource language modeling will bring AI to underserved regions
- On-device NLP (powered by quantized PyTorch models) will enable private, fast AI on smartphones
- Instruction-tuned models like ChatGPT will continue evolving, and many are trained using PyTorch
Conclusion: Start Your NLP Journey with PyTorch
Whether you’re analyzing product reviews or building the next intelligent assistant, PyTorch offers an accessible, flexible, and powerful platform for natural language processing.
With a growing ecosystem, strong support for state-of-the-art models, and seamless integration with real-world tools, PyTorch helps you go from beginner to NLP expert — one model at a time.
So if you’re serious about future-proofing your AI skills, there’s no better time to start learning PyTorch for NLP than now.
Sources That Inspired This Article
- PyTorch Official Documentation
- Hugging Face Transformers
- FastAI Text Tutorials
- Papers With Code – NLP Benchmarks
- DeepLearning.ai NLP Specialization
- Stanford CS224N: Natural Language Processing with Deep Learning
Website: https://4news.tech
Email: [email protected]